OpenID Connect (OIDC) Bearer token authentication
Secure HTTP access to Jakarta REST (formerly known as JAX-RS) endpoints in your application with Bearer token authentication by using the Quarkus OpenID Connect (OIDC) extension.
Overview of the Bearer token authentication mechanism in Quarkus
Quarkus supports the Bearer token authentication mechanism through the Quarkus OpenID Connect (OIDC) extension.
The bearer tokens are issued by OIDC and OAuth 2.0 compliant authorization servers, such as Keycloak.
Bearer token authentication is the process of authorizing HTTP requests based on the existence and validity of a bearer token. The bearer token provides information about the subject of the call, which is used to determine whether or not an HTTP resource can be accessed.
The following diagrams outline the Bearer token authentication mechanism in Quarkus:
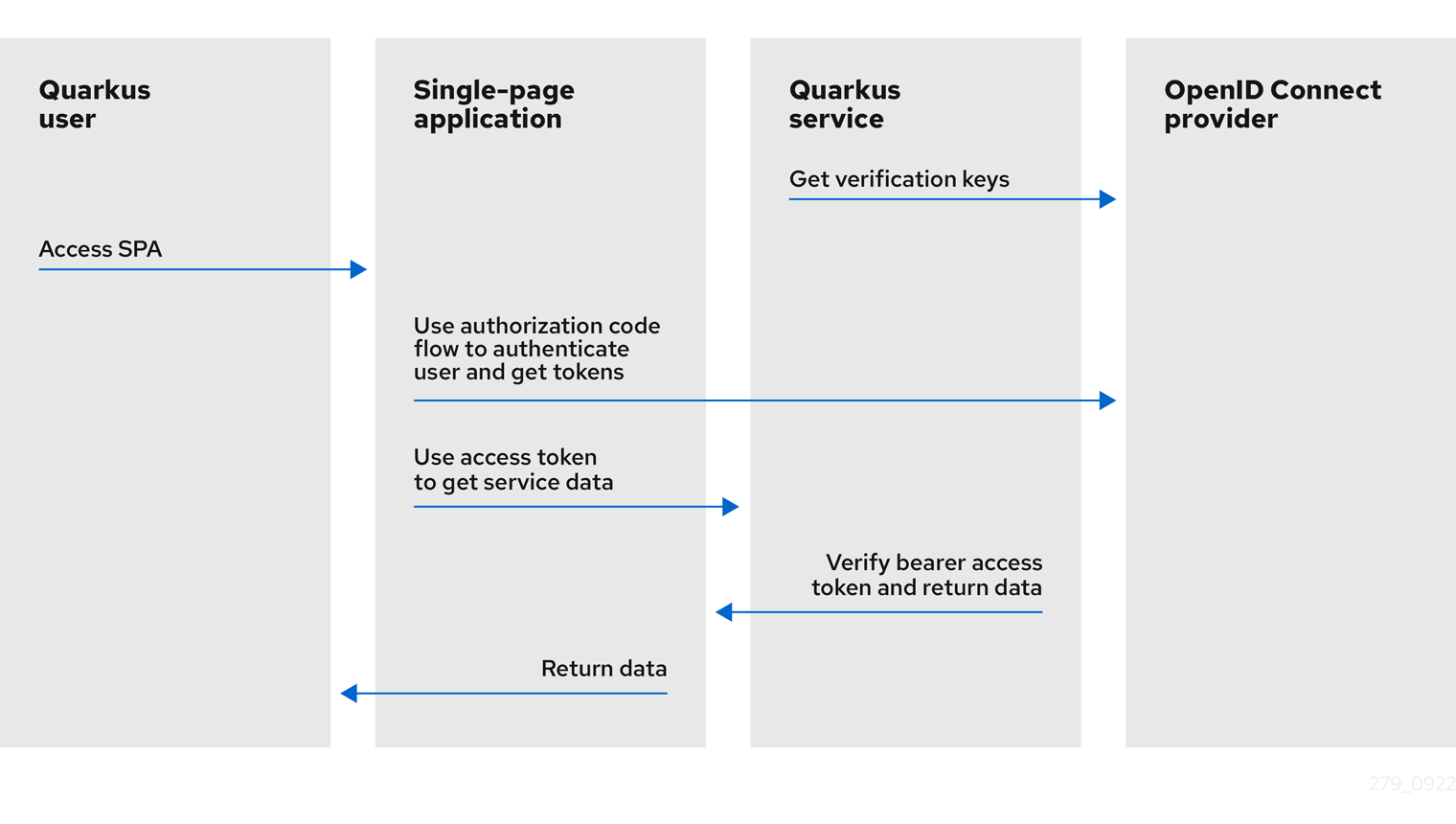
-
The Quarkus service retrieves verification keys from the OpenID Connect provider. The verification keys are used to verify the bearer access token signatures.
-
The Quarkus user accesses the Single-page application.
-
The Single-page application uses Authorization Code Flow to authenticate the user and retrieve tokens from the OpenID Connect provider.
-
The Single-page application uses the access token to retrieve the service data from the Quarkus service.
-
The Quarkus service verifies the bearer access token signature using the verification keys, checks the token expiry date and other claims, allows the request to proceed if the token is valid, and returns the service response to the Single-page application.
-
The Single-page application returns the same data to the Quarkus user.
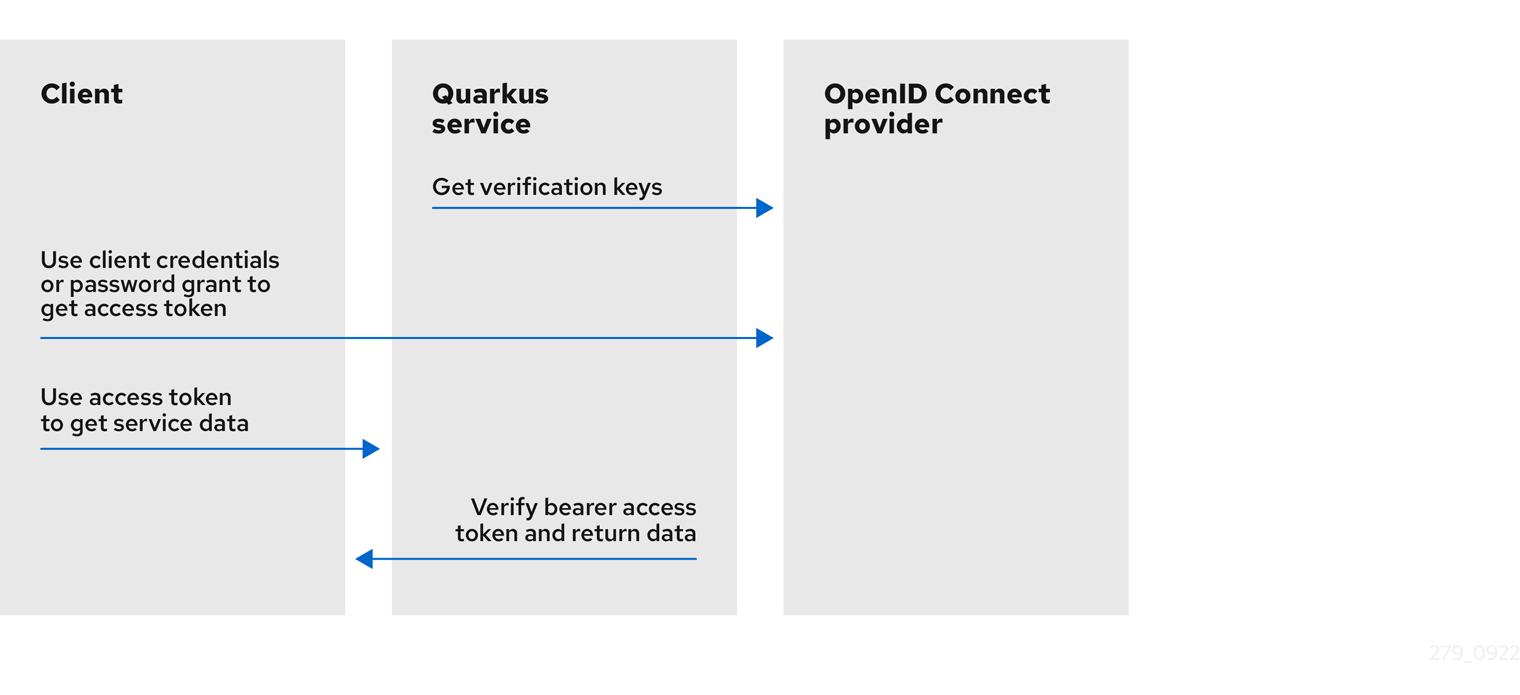
-
The Quarkus service retrieves verification keys from the OpenID Connect provider. The verification keys are used to verify the bearer access token signatures.
-
The Client uses
client_credentials
that requires client ID and secret or password grant, which also requires client ID, secret, user name, and password to retrieve the access token from the OpenID Connect provider. -
The Client uses the access token to retrieve the service data from the Quarkus service.
-
The Quarkus service verifies the bearer access token signature using the verification keys, checks the token expiry date and other claims, allows the request to proceed if the token is valid, and returns the service response to the Client.
If you need to authenticate and authorize the users using OpenID Connect Authorization Code Flow, see OIDC code flow mechanism for protecting web applications. Also, if you use Keycloak and bearer tokens, see Using Keycloak to Centralize Authorization.
To learn about how you can protect service applications by using OIDC Bearer token authentication, see OIDC Bearer token authentication tutorial.
If you want to protect web applications by using OIDC authorization code flow authentication, see OIDC authorization code flow authentication.
For information about how to support multiple tenants, see Using OpenID Connect Multi-Tenancy.
Accessing JWT claims
If you need to access JWT token claims then you can inject JsonWebToken
:
package org.acme.security.openid.connect;
import org.eclipse.microprofile.jwt.JsonWebToken;
import jakarta.inject.Inject;
import jakarta.annotation.security.RolesAllowed;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.core.MediaType;
@Path("/api/admin")
public class AdminResource {
@Inject
JsonWebToken jwt;
@GET
@RolesAllowed("admin")
@Produces(MediaType.TEXT_PLAIN)
public String admin() {
return "Access for subject " + jwt.getSubject() + " is granted";
}
}
Injection of JsonWebToken
is supported in @ApplicationScoped
, @Singleton
and @RequestScoped
scopes however the use of @RequestScoped
is required if the individual claims are injected as simple types, please see Support Injection Scopes for JsonWebToken and Claims for more details.
User Info
Set quarkus.oidc.authentication.user-info-required=true
if a UserInfo JSON object from the OIDC userinfo endpoint has to be requested.
A request will be sent to the OpenID Provider UserInfo endpoint and an io.quarkus.oidc.UserInfo
(a simple jakarta.json.JsonObject
wrapper) object will be created.
io.quarkus.oidc.UserInfo
can be either injected or accessed as a SecurityIdentity userinfo
attribute.
Configuration Metadata
The current tenant’s discovered OpenID Connect Configuration Metadata is represented by io.quarkus.oidc.OidcConfigurationMetadata
and can be either injected or accessed as a SecurityIdentity
configuration-metadata
attribute.
The default tenant’s OidcConfigurationMetadata
is injected if the endpoint is public.
Token Claims And SecurityIdentity Roles
SecurityIdentity roles can be mapped from the verified JWT access tokens as follows:
-
If
quarkus.oidc.roles.role-claim-path
property is set and matching array or string claims are found then the roles are extracted from these claims. For example,customroles
,customroles/array
,scope
,"http://namespace-qualified-custom-claim"/roles
,"http://namespace-qualified-roles"
, etc. -
If
groups
claim is available then its value is used -
If
realm_access/roles
orresource_access/client_id/roles
(whereclient_id
is the value of thequarkus.oidc.client-id
property) claim is available then its value is used. This check supports the tokens issued by Keycloak
If the token is opaque (binary) then a scope
property from the remote token introspection response will be used.
If UserInfo is the source of the roles then set quarkus.oidc.authentication.user-info-required=true
and quarkus.oidc.roles.source=userinfo
, and if needed, quarkus.oidc.roles.role-claim-path
.
Additionally, a custom SecurityIdentityAugmentor
can also be used to add the roles as documented in Security Identity Customization.
Token Verification And Introspection
If the token is a JWT token then, by default, it will be verified with a JsonWebKey
(JWK) key from a local JsonWebKeySet
retrieved from the OpenID Connect Provider’s JWK endpoint. The token’s key identifier kid
header value will be used to find the matching JWK key.
If no matching JWK
is available locally then JsonWebKeySet
will be refreshed by fetching the current key set from the JWK endpoint. The JsonWebKeySet
refresh can be repeated only after the quarkus.oidc.token.forced-jwk-refresh-interval
(default is 10 minutes) expires.
If no matching JWK
is available after the refresh then the JWT token will be sent to the OpenID Connect Provider’s token introspection endpoint.
If the token is opaque (it can be a binary token or an encrypted JWT token) then it will always be sent to the OpenID Connect Provider’s token introspection endpoint.
If you work with JWT tokens only and expect that a matching JsonWebKey
will always be available (possibly after a key set refresh) then you should disable the token introspection:
quarkus.oidc.token.allow-jwt-introspection=false
quarkus.oidc.token.allow-opaque-token-introspection=false
However, there could be cases where JWT tokens must be verified via the introspection only. It can be forced by configuring an introspection endpoint address only, for example, in case of Keycloak you can do it like this:
quarkus.oidc.auth-server-url=http://localhost:8180/realms/quarkus
quarkus.oidc.discovery-enabled=false
# Token Introspection endpoint: http://localhost:8180/realms/quarkus/protocol/openid-connect/tokens/introspect
quarkus.oidc.introspection-path=/protocol/openid-connect/tokens/introspect
An advantage of this indirect enforcement of JWT tokens being only introspected remotely is that two remote call are avoided: a remote OIDC metadata discovery call followed by another remote call fetching the verification keys which will not be used, while its disavantage is that the users need to know the introspection endpoint address and configure it manually.
The alternative approach is to allow discovering the OIDC metadata (which is a default option) but require that only the remote JWT introspection is performed:
quarkus.oidc.auth-server-url=http://localhost:8180/realms/quarkus
quarkus.oidc.token.require-jwt-introspection-only=true
An advantage of this approach is that the configuration is simple and easy to understand, while its disavantage is that a remote OIDC metadata discovery call is required to discover an introspection endpoint address (though the verification keys will also not be fetched).
Note that io.quarkus.oidc.TokenIntrospection
(a simple jakarta.json.JsonObject
wrapper) object will be created and can be either injected or accessed as a SecurityIdentity introspection
attribute if either JWT or opaque token has been successfully introspected.
Token Introspection and UserInfo Cache
All opaque and sometimes JWT Bearer access tokens have to be remotely introspected. If UserInfo
is also required then the same access token will be used to do a remote call to OpenID Connect Provider again. So, if UserInfo
is required and the current access token is opaque then for every such token there will be 2 remote calls done - one to introspect it and one to get UserInfo with it, and if the token is JWT then usually only a single remote call will be needed - to get UserInfo with it.
The cost of making up to 2 remote calls per every incoming bearer or code flow access token can sometimes be problematic.
If it is the case in your production then it can be recommended that the token introspection and UserInfo
data are cached for a short period of time, for example, for 3 or 5 minutes.
quarkus-oidc
provides quarkus.oidc.TokenIntrospectionCache
and quarkus.oidc.UserInfoCache
interfaces which can be used to implement @ApplicationScoped
cache implementation which can be used to store and retrieve quarkus.oidc.TokenIntrospection
and/or quarkus.oidc.UserInfo
objects, for example:
@ApplicationScoped
@Alternative
@Priority(1)
public class CustomIntrospectionUserInfoCache implements TokenIntrospectionCache, UserInfoCache {
...
}
Each OIDC tenant can either permit or deny storing its quarkus.oidc.TokenIntrospection
and/or quarkus.oidc.UserInfo
data with boolean quarkus.oidc."tenant".allow-token-introspection-cache
and quarkus.oidc."tenant".allow-user-info-cache
properties.
Additionally, quarkus-oidc
provides a simple default memory based token cache which implements both quarkus.oidc.TokenIntrospectionCache
and quarkus.oidc.UserInfoCache
interfaces.
It can be activated and configured as follows:
# 'max-size' is 0 by default so the cache can be activated by setting 'max-size' to a positive value.
quarkus.oidc.token-cache.max-size=1000
# 'time-to-live' specifies how long a cache entry can be valid for and will be used by a cleanup timer.
quarkus.oidc.token-cache.time-to-live=3M
# 'clean-up-timer-interval' is not set by default so the cleanup timer can be activated by setting 'clean-up-timer-interval'.
quarkus.oidc.token-cache.clean-up-timer-interval=1M
The default cache uses a token as a key and each entry can have TokenIntrospection
and/or UserInfo
. It will only keep up to a max-size
number of entries. If the cache is full when a new entry is to be added then an attempt will be made to find a space for it by removing a single expired entry. Additionally, the cleanup timer, if activated, will periodically check for the expired entries and remove them.
Please experiment with the default cache implementation or register a custom one.
JSON Web Token Claim Verification
Once the bearer JWT token’s signature has been verified and its expires at
(exp
) claim has been checked, the iss
(issuer
) claim value is verified next.
By default, the iss
claim value is compared to the issuer
property which may have been discovered in the well-known provider configuration.
But if quarkus.oidc.token.issuer
property is set then the iss
claim value is compared to it instead.
In some cases, this iss
claim verification may not work. For example, if the discovered issuer
property contains an internal HTTP/IP address while the token iss
claim value contains an external HTTP/IP address. Or when a discovered issuer
property contains the template tenant variable but the token iss
claim value has the complete tenant-specific issuer value.
In such cases you may want to consider skipping the issuer verification by setting quarkus.oidc.token.issuer=any
. Please note that it is not recommended and should be avoided unless no other options are available:
-
If you work with Keycloak and observe the issuer verification errors due to the different host addresses then configure Keycloak with a
KEYCLOAK_FRONTEND_URL
property to ensure the same host address is used. -
If the
iss
property is tenant specific in a multi-tenant deployment then you can use theSecurityIdentity
tenant-id
attribute to check the issuer is correct in the endpoint itself or the custom Jakarta REST filter, for example:
import jakarta.inject.Inject;
import jakarta.ws.rs.container.ContainerRequestContext;
import jakarta.ws.rs.container.ContainerRequestFilter;
import jakarta.ws.rs.ext.Provider;
import org.eclipse.microprofile.jwt.JsonWebToken;
import io.quarkus.oidc.OidcConfigurationMetadata;
import io.quarkus.security.identity.SecurityIdentity;
@Provider
public class IssuerValidator implements ContainerRequestFilter {
@Inject
OidcConfigurationMetadata configMetadata;
@Inject JsonWebToken jwt;
@Inject SecurityIdentity identity;
public void filter(ContainerRequestContext requestContext) {
String issuer = configMetadata.getIssuer().replace("{tenant-id}", identity.getAttribute("tenant-id"));
if (!issuer.equals(jwt.getIssuer())) {
requestContext.abortWith(Response.status(401).build());
}
}
}
Note it is also recommended to use quarkus.oidc.token.audience
property to verify the token aud
(audience
) claim value.
Single Page Applications
Single Page Application (SPA) typically uses XMLHttpRequest
(XHR) and the JavaScript utility code provided by the OpenID Connect provider to acquire a bearer token and use it
to access Quarkus service
applications.
For example, here is how you can use keycloak.js
to authenticate the users and refresh the expired tokens from the SPA:
<html>
<head>
<title>keycloak-spa</title>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script src="http://localhost:8180/js/keycloak.js"></script>
<script>
var keycloak = new Keycloak();
keycloak.init({onLoad: 'login-required'}).success(function () {
console.log('User is now authenticated.');
}).error(function () {
window.location.reload();
});
function makeAjaxRequest() {
axios.get("/api/hello", {
headers: {
'Authorization': 'Bearer ' + keycloak.token
}
})
.then( function (response) {
console.log("Response: ", response.status);
}).catch(function (error) {
console.log('refreshing');
keycloak.updateToken(5).then(function () {
console.log('Token refreshed');
}).catch(function () {
console.log('Failed to refresh token');
window.location.reload();
});
});
}
</script>
</head>
<body>
<button onclick="makeAjaxRequest()">Request</button>
</body>
</html>
Cross Origin Resource Sharing
If you plan to consume your OpenID Connect service
application from a Single Page Application running on a different domain, you will need to configure CORS (Cross-Origin Resource Sharing). Please read the HTTP CORS documentation for more details.
Provider Endpoint configuration
OIDC service
application needs to know OpenID Connect provider’s token, JsonWebKey
(JWK) set and possibly UserInfo
and introspection endpoint addresses.
By default, they are discovered by adding a /.well-known/openid-configuration
path to the configured quarkus.oidc.auth-server-url
.
Alternatively, if the discovery endpoint is not available, or if you would like to save on the discovery endpoint round-trip, you can disable the discovery and configure them with relative path values, for example:
quarkus.oidc.auth-server-url=http://localhost:8180/realms/quarkus
quarkus.oidc.discovery-enabled=false
# Token endpoint: http://localhost:8180/realms/quarkus/protocol/openid-connect/token
quarkus.oidc.token-path=/protocol/openid-connect/token
# JWK set endpoint: http://localhost:8180/realms/quarkus/protocol/openid-connect/certs
quarkus.oidc.jwks-path=/protocol/openid-connect/certs
# UserInfo endpoint: http://localhost:8180/realms/quarkus/protocol/openid-connect/userinfo
quarkus.oidc.user-info-path=/protocol/openid-connect/userinfo
# Token Introspection endpoint: http://localhost:8180/realms/quarkus/protocol/openid-connect/tokens/introspect
quarkus.oidc.introspection-path=/protocol/openid-connect/tokens/introspect
Token Propagation
Please see Token Propagation section about the Bearer access token propagation to the downstream services.
Oidc Provider Client Authentication
quarkus.oidc.runtime.OidcProviderClient
is used when a remote request to an OpenID Connect Provider has to be done. If the bearer token has to be introspected then OidcProviderClient
has to authenticate to the OpenID Connect Provider. Please see OidcProviderClient Authentication for more information about all the supported authentication options.
Testing
Start by adding the following dependencies to your test project:
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-junit5</artifactId>
<scope>test</scope>
</dependency>
testImplementation("io.rest-assured:rest-assured")
testImplementation("io.quarkus:quarkus-junit5")
Wiremock
Add the following dependencies to your test project:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-test-oidc-server</artifactId>
<scope>test</scope>
</dependency>
testImplementation("io.quarkus:quarkus-test-oidc-server")
Prepare the REST test endpoint, set application.properties
, for example:
# keycloak.url is set by OidcWiremockTestResource
quarkus.oidc.auth-server-url=${keycloak.url}/realms/quarkus/
quarkus.oidc.client-id=quarkus-service-app
quarkus.oidc.application-type=service
and finally write the test code, for example:
import static org.hamcrest.Matchers.equalTo;
import java.util.Set;
import org.junit.jupiter.api.Test;
import io.quarkus.test.common.QuarkusTestResource;
import io.quarkus.test.junit.QuarkusTest;
import io.quarkus.test.oidc.server.OidcWiremockTestResource;
import io.restassured.RestAssured;
import io.smallrye.jwt.build.Jwt;
@QuarkusTest
@QuarkusTestResource(OidcWiremockTestResource.class)
public class BearerTokenAuthorizationTest {
@Test
public void testBearerToken() {
RestAssured.given().auth().oauth2(getAccessToken("alice", Set.of("user")))
.when().get("/api/users/me")
.then()
.statusCode(200)
// the test endpoint returns the name extracted from the injected SecurityIdentity Principal
.body("userName", equalTo("alice"));
}
private String getAccessToken(String userName, Set<String> groups) {
return Jwt.preferredUserName(userName)
.groups(groups)
.issuer("https://server.example.com")
.audience("https://service.example.com")
.sign();
}
}
Note that the quarkus-test-oidc-server
extension includes a signing RSA private key file in a JSON Web Key
(JWK
) format and points to it with a smallrye.jwt.sign.key.location
configuration property. It allows to use a no argument sign()
operation to sign the token.
Testing your quarkus-oidc
service
application with OidcWiremockTestResource
provides the best coverage as even the communication channel is tested against the Wiremock HTTP stubs.
OidcWiremockTestResource
will be enhanced going forward to support more complex bearer token test scenarios.
If there is an immediate need for a test to define Wiremock stubs not currently supported by OidcWiremockTestResource
one can do so via a WireMockServer
instance injected into the test class, for example:
|
package io.quarkus.it.keycloak;
import static com.github.tomakehurst.wiremock.client.WireMock.matching;
import static org.hamcrest.Matchers.equalTo;
import org.junit.jupiter.api.Test;
import com.github.tomakehurst.wiremock.WireMockServer;
import com.github.tomakehurst.wiremock.client.WireMock;
import io.quarkus.test.junit.QuarkusTest;
import io.quarkus.test.oidc.server.OidcWireMock;
import io.restassured.RestAssured;
@QuarkusTest
public class CustomOidcWireMockStubTest {
@OidcWireMock
WireMockServer wireMockServer;
@Test
public void testInvalidBearerToken() {
wireMockServer.stubFor(WireMock.post("/auth/realms/quarkus/protocol/openid-connect/token/introspect")
.withRequestBody(matching(".*token=invalid_token.*"))
.willReturn(WireMock.aResponse().withStatus(400)));
RestAssured.given().auth().oauth2("invalid_token").when()
.get("/api/users/me/bearer")
.then()
.statusCode(401)
.header("WWW-Authenticate", equalTo("Bearer"));
}
}
Dev Services for Keycloak
Using Dev Services for Keycloak is recommended for the integration testing against Keycloak.
Dev Services for Keycloak
will launch and initialize a test container: it will create a quarkus
realm, a quarkus-app
client (secret
secret) and add alice
(admin
and user
roles) and bob
(user
role) users, where all of these properties can be customized.
First you need to add the following dependency:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-test-keycloak-server</artifactId>
<scope>test</scope>
</dependency>
testImplementation("io.quarkus:quarkus-test-keycloak-server")
which provides a utility class io.quarkus.test.keycloak.client.KeycloakTestClient
you can use in tests for acquiring the access tokens.
Next prepare your application.properties
. You can start with a completely empty application.properties
as Dev Services for Keycloak
will register quarkus.oidc.auth-server-url
pointing to the running test container as well as quarkus.oidc.client-id=quarkus-app
and quarkus.oidc.credentials.secret=secret
.
But if you already have all the required quarkus-oidc
properties configured then you only need to associate quarkus.oidc.auth-server-url
with the prod
profile for `Dev Services for Keycloak`to start a container, for example:
%prod.quarkus.oidc.auth-server-url=http://localhost:8180/realms/quarkus
If a custom realm file has to be imported into Keycloak before running the tests then you can configure Dev Services for Keycloak
as follows:
%prod.quarkus.oidc.auth-server-url=http://localhost:8180/realms/quarkus
quarkus.keycloak.devservices.realm-path=quarkus-realm.json
Finally, write your test which will be executed in JVM mode:
package org.acme.security.openid.connect;
import io.quarkus.test.junit.QuarkusTest;
import io.quarkus.test.keycloak.client.KeycloakTestClient;
import io.restassured.RestAssured;
import org.junit.jupiter.api.Test;
@QuarkusTest
public class BearerTokenAuthenticationTest {
KeycloakTestClient keycloakClient = new KeycloakTestClient();
@Test
public void testAdminAccess() {
RestAssured.given().auth().oauth2(getAccessToken("alice"))
.when().get("/api/admin")
.then()
.statusCode(200);
RestAssured.given().auth().oauth2(getAccessToken("bob"))
.when().get("/api/admin")
.then()
.statusCode(403);
}
protected String getAccessToken(String userName) {
return keycloakClient.getAccessToken(userName);
}
}
and in native mode:
package org.acme.security.openid.connect;
import io.quarkus.test.junit.QuarkusIntegrationTest;
@QuarkusIntegrationTest
public class NativeBearerTokenAuthenticationIT extends BearerTokenAuthenticationTest {
}
Please see Dev Services for Keycloak for more information about the way it is initialized and configured.
KeycloakTestResourceLifecycleManager
If you need to do some integration testing against Keycloak then you are encouraged to do it with Dev Services For Keycloak.
Use KeycloakTestResourceLifecycleManager
for your tests only if there is a good reason not to use Dev Services for Keycloak
.
Start with adding the following dependency:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-test-keycloak-server</artifactId>
<scope>test</scope>
</dependency>
testImplementation("io.quarkus:quarkus-test-keycloak-server")
which provides io.quarkus.test.keycloak.server.KeycloakTestResourceLifecycleManager
- an implementation of io.quarkus.test.common.QuarkusTestResourceLifecycleManager
which starts a Keycloak container.
And configure the Maven Surefire plugin as follows:
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<systemPropertyVariables>
<!-- or, alternatively, configure 'keycloak.version' -->
<keycloak.docker.image>${keycloak.docker.image}</keycloak.docker.image>
<!--
Disable HTTPS if required:
<keycloak.use.https>false</keycloak.use.https>
-->
</systemPropertyVariables>
</configuration>
</plugin>
(and similarly maven.failsafe.plugin
when testing in native image).
Prepare the REST test endpoint, set application.properties
, for example:
# keycloak.url is set by KeycloakTestResourceLifecycleManager
quarkus.oidc.auth-server-url=${keycloak.url}/realms/quarkus/
quarkus.oidc.client-id=quarkus-service-app
quarkus.oidc.credentials=secret
quarkus.oidc.application-type=service
and finally write the test code, for example:
import static io.quarkus.test.keycloak.server.KeycloakTestResourceLifecycleManager.getAccessToken;
import static org.hamcrest.Matchers.equalTo;
import org.hamcrest.Matchers;
import org.junit.jupiter.api.Test;
import io.quarkus.test.common.QuarkusTestResource;
import io.quarkus.test.junit.QuarkusTest;
import io.quarkus.test.keycloak.server.KeycloakTestResourceLifecycleManager;
import io.restassured.RestAssured;
@QuarkusTest
@QuarkusTestResource(KeycloakTestResourceLifecycleManager.class)
public class BearerTokenAuthorizationTest {
@Test
public void testBearerToken() {
RestAssured.given().auth().oauth2(getAccessToken("alice"))))
.when().get("/api/users/preferredUserName")
.then()
.statusCode(200)
// the test endpoint returns the name extracted from the injected SecurityIdentity Principal
.body("userName", equalTo("alice"));
}
}
KeycloakTestResourceLifecycleManager
registers alice
and admin
users. The user alice
has the user
role only by default - it can be customized with a keycloak.token.user-roles
system property. The user admin
has the user
and admin
roles by default - it can be customized with a keycloak.token.admin-roles
system property.
By default, KeycloakTestResourceLifecycleManager
uses HTTPS to initialize a Keycloak instance which can be disabled with keycloak.use.https=false
.
Default realm name is quarkus
and client id - quarkus-service-app
- set keycloak.realm
and keycloak.service.client
system properties to customize the values if needed.
Local Public Key
You can also use a local inlined public key for testing your quarkus-oidc
service
applications:
quarkus.oidc.client-id=test
quarkus.oidc.public-key=MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAlivFI8qB4D0y2jy0CfEqFyy46R0o7S8TKpsx5xbHKoU1VWg6QkQm+ntyIv1p4kE1sPEQO73+HY8+Bzs75XwRTYL1BmR1w8J5hmjVWjc6R2BTBGAYRPFRhor3kpM6ni2SPmNNhurEAHw7TaqszP5eUF/F9+KEBWkwVta+PZ37bwqSE4sCb1soZFrVz/UT/LF4tYpuVYt3YbqToZ3pZOZ9AX2o1GCG3xwOjkc4x0W7ezbQZdC9iftPxVHR8irOijJRRjcPDtA6vPKpzLl6CyYnsIYPd99ltwxTHjr3npfv/3Lw50bAkbT4HeLFxTx4flEoZLKO/g0bAoV2uqBhkA9xnQIDAQAB
smallrye.jwt.sign.key.location=/privateKey.pem
copy privateKey.pem
from the integration-tests/oidc-tenancy
in the main
Quarkus repository and use a test code similar to the one in the Wiremock
section above to generate JWT tokens. You can use your own test keys if preferred.
This approach provides a more limited coverage compared to the Wiremock approach - for example, the remote communication code is not covered.
TestSecurity annotation
You can use @TestSecurity
and @OidcSecurity
annotations for testing the service
application endpoint code which depends on the injected JsonWebToken
as well as UserInfo
and OidcConfigurationMetadata
.
Add the following dependency:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-test-security-oidc</artifactId>
<scope>test</scope>
</dependency>
testImplementation("io.quarkus:quarkus-test-security-oidc")
and write a test code like this one:
import static org.hamcrest.Matchers.is;
import org.junit.jupiter.api.Test;
import io.quarkus.test.common.http.TestHTTPEndpoint;
import io.quarkus.test.junit.QuarkusTest;
import io.quarkus.test.security.TestSecurity;
import io.quarkus.test.security.oidc.Claim;
import io.quarkus.test.security.oidc.ConfigMetadata;
import io.quarkus.test.security.oidc.OidcSecurity;
import io.quarkus.test.security.oidc.OidcConfigurationMetadata;
import io.quarkus.test.security.oidc.UserInfo;
import io.restassured.RestAssured;
@QuarkusTest
@TestHTTPEndpoint(ProtectedResource.class)
public class TestSecurityAuthTest {
@Test
@TestSecurity(user = "userOidc", roles = "viewer")
public void testOidc() {
RestAssured.when().get("test-security-oidc").then()
.body(is("userOidc:viewer"));
}
@Test
@TestSecurity(user = "userOidc", roles = "viewer")
@OidcSecurity(claims = {
@Claim(key = "email", value = "user@gmail.com")
}, userinfo = {
@UserInfo(key = "sub", value = "subject")
}, config = {
@ConfigMetadata(key = "issuer", value = "issuer")
})
public void testOidcWithClaimsUserInfoAndMetadata() {
RestAssured.when().get("test-security-oidc-claims-userinfo-metadata").then()
.body(is("userOidc:viewer:user@gmail.com:subject:issuer"));
}
}
where ProtectedResource
class may look like this:
import io.quarkus.oidc.OidcConfigurationMetadata;
import io.quarkus.oidc.UserInfo;
import org.eclipse.microprofile.jwt.JsonWebToken;
@Path("/service")
@Authenticated
public class ProtectedResource {
@Inject
JsonWebToken accessToken;
@Inject
UserInfo userInfo;
@Inject
OidcConfigurationMetadata configMetadata;
@GET
@Path("test-security-oidc")
public String testSecurityOidc() {
return accessToken.getName() + ":" + accessToken.getGroups().iterator().next();
}
@GET
@Path("test-security-oidc-claims-userinfo-metadata")
public String testSecurityOidcWithClaimsUserInfoMetadata() {
return accessToken.getName() + ":" + accessToken.getGroups().iterator().next()
+ ":" + accessToken.getClaim("email")
+ ":" + userInfo.getString("sub")
+ ":" + configMetadata.get("issuer");
}
}
Note that @TestSecurity
annotation must always be used and its user
property is returned as JsonWebToken.getName()
and roles
property - as JsonWebToken.getGroups()
.
@OidcSecurity
annotation is optional and can be used to set the additional token claims, as well as UserInfo
and OidcConfigurationMetadata
properties.
Additionally, if quarkus.oidc.token.issuer
property is configured then it will be used as an OidcConfigurationMetadata
issuer
property value.
If you work with the opaque tokens then you can test them as follows:
import static org.hamcrest.Matchers.is;
import org.junit.jupiter.api.Test;
import io.quarkus.test.common.http.TestHTTPEndpoint;
import io.quarkus.test.junit.QuarkusTest;
import io.quarkus.test.security.TestSecurity;
import io.quarkus.test.security.oidc.OidcSecurity;
import io.quarkus.test.security.oidc.TokenIntrospection;
import io.restassured.RestAssured;
@QuarkusTest
@TestHTTPEndpoint(ProtectedResource.class)
public class TestSecurityAuthTest {
@Test
@TestSecurity(user = "userOidc", roles = "viewer")
@OidcSecurity(introspectionRequired = true,
introspection = {
@TokenIntrospection(key = "email", value = "user@gmail.com")
}
)
public void testOidcWithClaimsUserInfoAndMetadata() {
RestAssured.when().get("test-security-oidc-claims-userinfo-metadata").then()
.body(is("userOidc:viewer:userOidc:viewer"));
}
}
where ProtectedResource
class may look like this:
import io.quarkus.oidc.TokenIntrospection;
import io.quarkus.security.identity.SecurityIdentity;
@Path("/service")
@Authenticated
public class ProtectedResource {
@Inject
SecurityIdentity securityIdentity;
@Inject
TokenIntrospection introspection;
@GET
@Path("test-security-oidc-opaque-token")
public String testSecurityOidcOpaqueToken() {
return securityIdentity.getPrincipal().getName() + ":" + securityIdentity.getRoles().iterator().next()
+ ":" + introspection.getString("username")
+ ":" + introspection.getString("scope")
+ ":" + introspection.getString("email");
}
}
Note that @TestSecurity
user
and roles
attributes are available as TokenIntrospection
username
and scope
properties and you can use io.quarkus.test.security.oidc.TokenIntrospection
to add the additional introspection response properties such as an email
, etc.
This is particularly useful if the same set of security settings needs to be used in multiple test methods. |
How to check the errors in the logs
Please enable io.quarkus.oidc.runtime.OidcProvider
TRACE
level logging to see more details about the token verification errors:
quarkus.log.category."io.quarkus.oidc.runtime.OidcProvider".level=TRACE
quarkus.log.category."io.quarkus.oidc.runtime.OidcProvider".min-level=TRACE
Please enable io.quarkus.oidc.runtime.OidcRecorder
TRACE
level logging to see more details about the OidcProvider client initialization errors:
quarkus.log.category."io.quarkus.oidc.runtime.OidcRecorder".level=TRACE
quarkus.log.category."io.quarkus.oidc.runtime.OidcRecorder".min-level=TRACE
External and Internal Access to OpenID Connect Provider
Note that the OpenID Connect Provider externally accessible token and other endpoints may have different HTTP(S) URLs compared to the URLs auto-discovered or configured relative to quarkus.oidc.auth-server-url
internal URL. For example, if your SPA acquires a token from an external token endpoint address and sends it to Quarkus as a bearer token then an issuer verification failure may be reported by the endpoint.
In such cases, if you work with Keycloak then please start it with a KEYCLOAK_FRONTEND_URL
system property set to the externally accessible base URL.
If you work with other Openid Connect providers then please check your provider’s documentation.
How to use 'client-id' property
quarkus.oidc.client-id
property identifies an OpenID Connect Client which requested the current bearer token. It can be an SPA application running in a browser or a Quarkus web-app
confidential client application propagating the access token to the Quarkus service
application.
This property is required if the service
application is expected to introspect the tokens remotely - which is always the case for the opaque tokens.
This property is optional if the local Json Web Key token verification only is used.
Nonetheless, setting this property is encouraged even if the endpoint does not require access to the remote introspection endpoint. The reasons behind it that client-id
, if set, can be used to verify the token audience and will also be included in the logs when the token verification fails for the better traceability of the tokens issued to specific clients to be analyzed over a longer period of time.
For example, if your OpenID Connect provider sets a token audience then the following configuration pattern is recommended:
# Set client-id
quarkus.oidc.client-id=quarkus-app
# Token audience claim must contain 'quarkus-app'
quarkus.oidc.token.audience=${quarkus.oidc.client-id}
If you set quarkus.oidc.client-id
but your endpoint does not require remote access to one of OpenID Connect Provider endpoints (introspection, token acquisition, etc.) then do not set a client secret with the quarkus.oidc.credentials
or similar properties as it will not be used.
Note Quarkus web-app
applications always require quarkus.oidc.client-id
property.